Sunday, June 1, 2014
Sunday, May 25, 2014
this keyword in Java
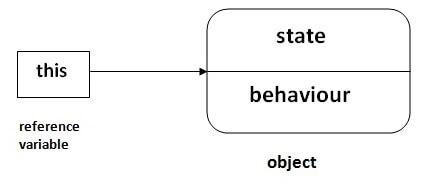
Here is given the usage of this keyword.
From - www.DreamsCoder.com
Wednesday, May 14, 2014
Star Pattern Program in C
/*Star Pattern Program in C
Author - Chinmay Mahajan*/
#include<stdio.h>
#include<conio.h>
void main()
{
int i,j,k,m,o,f,n;
clrscr();
printf("Enter value of n \n");
scanf("%d",&n);
k=n;
o=1;
for(i=0;i<n;i++) //for number of lines
{
for(j=0;j<k;j++) //for left spacing
{
printf("_");
}
for(m=0;m<o;m++) //for Printing *
{
printf(" *");
}
for(f=0;f<k;f++) //for right spacing
{
printf("_");
}
printf("\n"); //new line
k--;
o++;
}//outer for
}//main
Sunday, April 20, 2014
Socket Programming with File Handling
/*Client Socket program that accepts file name from Client and send it to Server, Server Side Socket reads the Content of a file and display them onto the Client Socket*/
/*Server Program (severprac.java)*/
import java.io.*;
import java.net.*;
public class serverprac
{
public static void main(String[] args) {
ServerSocket ss;
Socket client;
String nm;
try
{
ss = new ServerSocket(1212);
System.out.println("Server is Waitng For Client");
client = ss.accept();
System.out.println("Client is Connected");
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
BufferedReader br1 = new BufferedReader(new InputStreamReader(client.getInputStream()));
PrintStream ps = new PrintStream(client.getOutputStream());
String filenm = br1.readLine();
File f = new File(".");
File[] flist = f.listFiles();
for(File n:flist)
{
nm = n.getName();
if(nm.equals(filenm))
{
System.out.println("File is Present");
FileReader fr = new FileReader(nm);
BufferedReader bfr = new BufferedReader(fr);
String lne;
while(true)
{
lne = bfr.readLine();
if (lne == null)
break;
//System.out.println(lne);
ps.println(lne);
}
}
}
ps.close();
br1.close();
br.close();
ss.close();
}//try
catch(Exception e)
{
System.out.println(e);
}
}//main
}
/*Client Program (clientprac.java)*/
import java.io.*;import java.net.*;
public class clientprac
{
public static void main(String[] args)
{
Socket s;
try
{
s = new Socket("127.0.0.1",1212);
System.out.println("Clent is Conneccted to the Server");
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
BufferedReader br1 = new BufferedReader(new InputStreamReader(s.getInputStream()));
PrintStream ps = new PrintStream(s.getOutputStream());
System.out.println("Enter File name");
ps.println(br.readLine());
while(br1.readLine() !=null)
System.out.println(br1.readLine());
br1.close();
ps.close();
br.close();
br1.close();
s.close();
}
catch(Exception e)
{
System.out.println(""+e);
}//catch
}//main
}
Saturday, March 29, 2014
Inter - Thread communication using wait(), notify() and notify all() method in Java
/*
Write a program to illustrate inter - thread communication using wait(), notify() and notify all() method
*/
import java.io.*;
class q
{
String msg;
boolean flag;
q()
{
flag=false;
}
synchronized void get()
{
if(flag==false)
{
try
{
wait();
}//try
catch(InterruptedException e)
{
}//catch
}
flag=false;
System.out.println("Received Message"+msg);
notify();
}
synchronized void put(String x)
{
if(flag==true)
{
try
{
wait();
}
catch(InterruptedException e)
{
}//catch
}
msg = x;
flag = true;
if(msg.equalsIgnoreCase("Good Bye"))
System.exit(0);
notify();
}
}
class sender extends Thread{
q q1;
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
String s;
sender(q x)
{
q1 = x;
start();
}
public void run()
{
while(true)
{
System.out.println("Enter Message ");
try
{
s = br.readLine();
q1.put(s);
}
catch(IOException e)
{
}//catch
}//while
}//run
}
class receiver extends Thread
{
q q2;
receiver(q x)
{
q2 = x;
start();
}
public void run()
{
while(true)
{
q2.get();
}
}
}
class fourteen
{
public static void main(String args[])
{
q q4 = new q();
sender s = new sender(q4);
receiver r = new receiver(q4);
}
}
Write a program to illustrate inter - thread communication using wait(), notify() and notify all() method
*/
import java.io.*;
class q
{
String msg;
boolean flag;
q()
{
flag=false;
}
synchronized void get()
{
if(flag==false)
{
try
{
wait();
}//try
catch(InterruptedException e)
{
}//catch
}
flag=false;
System.out.println("Received Message"+msg);
notify();
}
synchronized void put(String x)
{
if(flag==true)
{
try
{
wait();
}
catch(InterruptedException e)
{
}//catch
}
msg = x;
flag = true;
if(msg.equalsIgnoreCase("Good Bye"))
System.exit(0);
notify();
}
}
class sender extends Thread{
q q1;
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
String s;
sender(q x)
{
q1 = x;
start();
}
public void run()
{
while(true)
{
System.out.println("Enter Message ");
try
{
s = br.readLine();
q1.put(s);
}
catch(IOException e)
{
}//catch
}//while
}//run
}
class receiver extends Thread
{
q q2;
receiver(q x)
{
q2 = x;
start();
}
public void run()
{
while(true)
{
q2.get();
}
}
}
class fourteen
{
public static void main(String args[])
{
q q4 = new q();
sender s = new sender(q4);
receiver r = new receiver(q4);
}
}
User Defined Exception Example in Java
/*
Write a class Student with attributes roll no, name, age and course. Initialize values through parameterized constructor. If age of student is not in between 15 and 21 then generate user-defined exception “Age Not Within The Range”.If name contains numbers or special symbols raise exception “Name not valid”
*/
import java.io.*;
class AgeNotWithinRangeException extends Exception
{
AgeNotWithinRangeException()
{
super();
}
}
class NameNotValidException extends Exception
{
NameNotValidException()
{
super();
}
}
class Student
{
private
int roll,age;
String name,course;
public
Student(int r,String nm,int ag,String co)
{
roll=r;
name=nm;
age=ag;
course=co;
System.out.println("Roll Number is "+roll);
System.out.println("Name is "+name);;
System.out.println("Age is "+age);
System.out.println("Course is "+course);
}
public static void main(String args[]) throws IOException
{
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int roll,age;
String name,course;
System.out.println("Enter No. :");
roll = Integer.parseInt(br.readLine());
System.out.println("Enter Name :");
name = br.readLine();
try
{
for(int i=0;i<name.length();i++)
{
char c = name.charAt(i);
if((c<65 || c>90) && (c<97 || c>122) && (c!=32))
{
throw new NameNotValidException();
}//if
}//for
}//try
catch(NameNotValidException n)
{
System.out.println("Invalid Name"+n);
}
System.out.println("Enter Age :");
age = Integer.parseInt(br.readLine());
try
{
if(age<15 || age>21)
{
throw new AgeNotWithinRangeException();
}
}//try
catch(AgeNotWithinRangeException e)
{
System.out.println("Invalid Age"+e);
}
System.out.println("Enter Course :");
course = br.readLine();
Student s = new Student(roll,name,age,course);
}
}
Write a class Student with attributes roll no, name, age and course. Initialize values through parameterized constructor. If age of student is not in between 15 and 21 then generate user-defined exception “Age Not Within The Range”.If name contains numbers or special symbols raise exception “Name not valid”
*/
import java.io.*;
class AgeNotWithinRangeException extends Exception
{
AgeNotWithinRangeException()
{
super();
}
}
class NameNotValidException extends Exception
{
NameNotValidException()
{
super();
}
}
class Student
{
private
int roll,age;
String name,course;
public
Student(int r,String nm,int ag,String co)
{
roll=r;
name=nm;
age=ag;
course=co;
System.out.println("Roll Number is "+roll);
System.out.println("Name is "+name);;
System.out.println("Age is "+age);
System.out.println("Course is "+course);
}
public static void main(String args[]) throws IOException
{
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int roll,age;
String name,course;
System.out.println("Enter No. :");
roll = Integer.parseInt(br.readLine());
System.out.println("Enter Name :");
name = br.readLine();
try
{
for(int i=0;i<name.length();i++)
{
char c = name.charAt(i);
if((c<65 || c>90) && (c<97 || c>122) && (c!=32))
{
throw new NameNotValidException();
}//if
}//for
}//try
catch(NameNotValidException n)
{
System.out.println("Invalid Name"+n);
}
System.out.println("Enter Age :");
age = Integer.parseInt(br.readLine());
try
{
if(age<15 || age>21)
{
throw new AgeNotWithinRangeException();
}
}//try
catch(AgeNotWithinRangeException e)
{
System.out.println("Invalid Age"+e);
}
System.out.println("Enter Course :");
course = br.readLine();
Student s = new Student(roll,name,age,course);
}
}
Subscribe to:
Posts (Atom)